It’s been a long time since I last blogged. In the interim I started a new job at Igalia as a JavaScript Engine Developer on the compilers team, and attended FOSDEM in Brussels several million years ago in early February back when “getting on a plane and traveling to a different country” was still a reasonable thing to do.
In this blog post I would like to present Temporal, a proposal to add modern and comprehensive handling of dates and times to the JavaScript language. This has been the project I’m working on at Igalia, as sponsored by Bloomberg. I’ve been working on it for the last 6 months, joining several of my coworkers in a cross-company group of talented people who have already been working on it for several years.
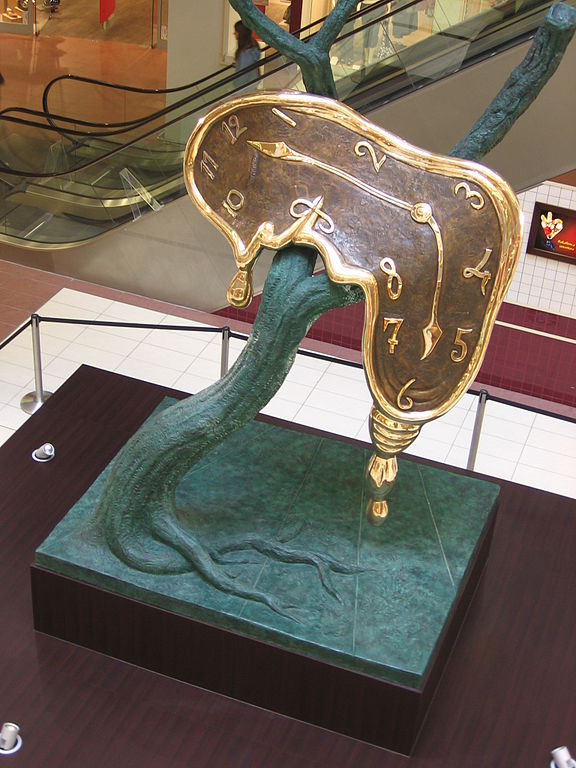
Date
… (Public domain photograph by Julo)I already collaborated on a blog post about Temporal, “Dates and Times in JavaScript”, so I won’t repeat all that here, but all the explanation you really need is that Temporal is a modern replacement for the Date
object in JavaScript, which is terrible. You may also want to read “Fixing JavaScript Date”, a two-part series providing further background, by Maggie Pint, one of the originators of Temporal.
How Temporal can be useful in GNOME
I’m aware that this blog is mostly read by the GNOME community. That’s why in this blog post I want to talk especially about how a large piece of desktop software like GNOME is affected by JavaScript Date
being so terrible.
Of course most improvements to the JavaScript language are driven by the needs of the web.1 But a few months ago this merge request caught my eye, fixing a bug that made the date displayed in GNOME wrong by a full 1,900 years! The difference between Date.getYear()
not doing what you expect (and Date.getFullYear()
doing it instead) is one of the really awful parts of JavaScript Date
. In this case if there had been a better API without evil traps, the mistake might not have been made in the first place, and it wouldn’t have come down to a last-minute code freeze break.
In the group working on the Temporal proposal we are seeking feedback from people who are willing to try out the Temporal API, so that we can find out if there are any parts that don’t meet people’s needs and change them before we try to move the proposal to Stage 3 of the TC39 process. Since I think GNOME Shell and GNOME Weather, and possibly other apps, might benefit from using this API when it becomes part of JavaScript in the future, I’d be interested in finding out what we in the GNOME community need from the Temporal API.
It seems to me the best way to do this would be to make a port of GNOME Shell and/or GNOME Weather to the experimental Temporal API, and see what issues come up. Unfortunately, it would defeat the purpose for me to do this myself, since I am already overly familiar with Temporal and by now its shortcomings are squarely in my blind spot! So instead I’ll offer my help and guidance to anyone who wants to try this out. Please get in touch with me if you are interested.
How to try it out
Since Temporal is of course not yet a built-in object in JavaScript, to try it out we will need to import a polyfill. We have published a polyfill which is experimental only, for the purpose of trying out the API and integrating it with existing code. Here’s a link to the API documentation.
The polyfill is primarily published as an NPM library, but we can get it to work with GJS quite easily. Here’s how I did it.
First I cloned the tc39/proposal-temporal repo, and ran npm install
and npm run build
in it. This generates a file called polyfill/script.js
which you can copy into your code, into a place in your imports path so that the importer can find it. Then you can import Temporal:
const {Temporal} = imports.temporal.temporal;
Note that the API is not stable, so only use this to try out the API and give feedback! Don’t actually include it in your code. We have every intention of changing the API, maybe even drastically, based on feedback that we receive.
Once you have tried it out, the easiest way to tell us about your findings is to complete the survey, but do also open an issue in the bug tracker if you have something specific.
Intl, or how to stop doing _("%B %-d %Y")
While I was browsing through GNOME Shell bug reports to find ones related to JavaScript Date
, I found several such as gnome-shell#2293 where the translated format strings lag behind the release while translators figure out how to translate cryptic strings such as "%B %-d %Y"
for their locales. By doing our own translations, we are actually creating the conditions to receive these kinds of bug reports in the first place. Translations for these kinds of formats that respect the formatting rules for each locale are already built into JavaScript engines nowadays, in the Intl API via libicu, and we could take advantage of these translations to take some pressure off of our translators.
In fact, we could do this right now already, no need to wait for the Temporal proposal to be adopted into JavaScript and subsequently make it into GJS. We already have everything we need in GNOME 3.36. With Intl, the function I linked above would become:
_updateTitle() {
const locale = getCachedLocale();
const timeSpanDay = GLib.TIME_SPAN_DAY / 1000;
const now = new Date();
const rtf = new Intl.RelativeTimeFormat(locale, {numeric: 'auto'});
if (this._startDate <= now && now <= this._endDate)
this._title.text = rtf.format(0, 'day');
else if (this._endDate < now && now - this._endDate < timeSpanDay)
this._title.text = rtf.format(-1, 'day');
else if (this._startDate > now && this._startDate - now < timeSpanDay)
this._title.text = rtf.format(1, 'day');
else if (this._startDate.getFullYear() === now.getFullYear())
this._title.text = this._startDate.toLocaleString(locale, {month: 'long', day: 'numeric'});
else
this._title.text = this._startDate.toLocaleString(locale, {year: 'numeric', month: 'long', day: 'numeric'});
}
(Note, this presumes a function getCachedLocale()
which determines the correct locale for Intl
by looking at the LC_TIME
, LC_ALL
, etc. evnvironment variables. If GNOME apps wanted to move to Intl generally, I think it might be worth adding such a function to GJS’s Gettext module.)
Whereas in the future with Temporal, it would be even simpler and clearer, and I couldn’t resist rewriting that method! We wouldn’t need to store a start Date
at 00:00 and end Date
at 23:59.999 which is really just a workaround for the fact that we are talking here about a date without a time component, that is purely a calendar day. Temporal covers this use case out of the box:
_updateTitle() {
const locale = getCachedLocale();
const today = Temporal.now.date();
const {days} = today.difference(this._date);
if (days <= 1) {
const rtf = new Intl.RelativeTimeFormat(locale, {numeric: 'auto'});
// Note: if this negation seems a bit unwieldy, be aware that we are
// considering revising the API to allow negative-valued durations
days = Temporal.Date.compare(today, this._date) < 0 ? days : -days;
this._title.text = rtf.format(days, 'day');
} else {
const options = {month: 'long', day: 'numeric'};
if (today.year !== this._date.year)
options.year = 'numeric';
this._title.text = this._date.toLocaleString(locale, options);
}
}
Calendar systems
One exciting thing about Temporal is that it will support non-Gregorian calendars. If you are a GNOME user or developer who uses a non-Gregorian calendar, or develops code for users who do, then please get in touch with me! In the group of people developing Temporal everyone uses the Gregorian calendar, so we have a knowledge gap about what users of other calendars need. We’d like to try to close this gap by talking to people.
A Final Note
In the past months I’ve not been much in the mood to write blog posts. My mind has been occupied worrying about the health of my family, friends, and myself; feeling fury and shame at the inequalities of our society that, frankly, the pandemic has made harder to fool ourselves into forgetting if it doesn’t affect us directly; and fury at our governments that perpetuate these problems and resist meaningful attempts at reform.
With all that’s going on in the world, blogging about technical achievements feels a bit ridiculous and inconsequential, but, well, I’m writing this, and you’re reading this, and here we are. So keep in mind there are other important things too. Be safe, be kind, but don’t forget to stay furious after the dust settles.
[1] One motivation for why some are eagerly awaiting Temporal as part of the JavaScript language, as opposed to a library, is that it would be built-in to the browser. The most popular library for fixing the deficiencies of Date
, moment.js, can mean an extra download of 20–100 kb, depending on whether you include all locales and support for time zones. This adds up to quite a lot of wasted data if you are downloading this on a large number of the websites you visit, but this specifically doesn’t affect GNOME. ↩